JavaScript 구글 Maps API 연동하여 지도 넣기 ( JavaScript 활용 )
페이지 정보

본문
구글 지도를 웹페이지에 삽입하는 방법입니다.
1. https://www.google.co.kr/maps/ 접속
2. 주소 또는 장소명을 검색
표시된 검색결과의 지도에서 원하는 위치를 마우스 클릭 하여 아래와 같이 " 이곳이 궁금한가요? " 클릭
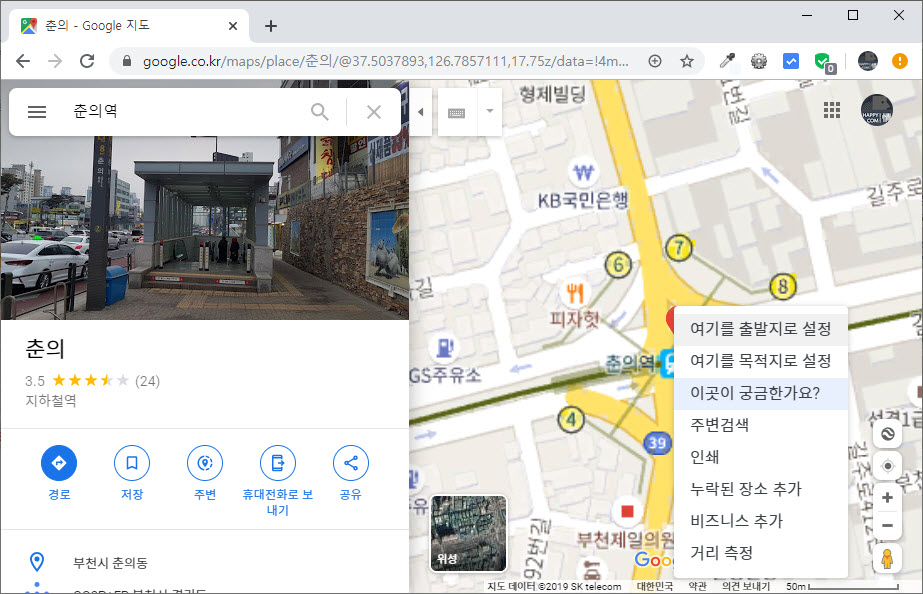
3. 화면 하단의 좌표를 확인합니다.
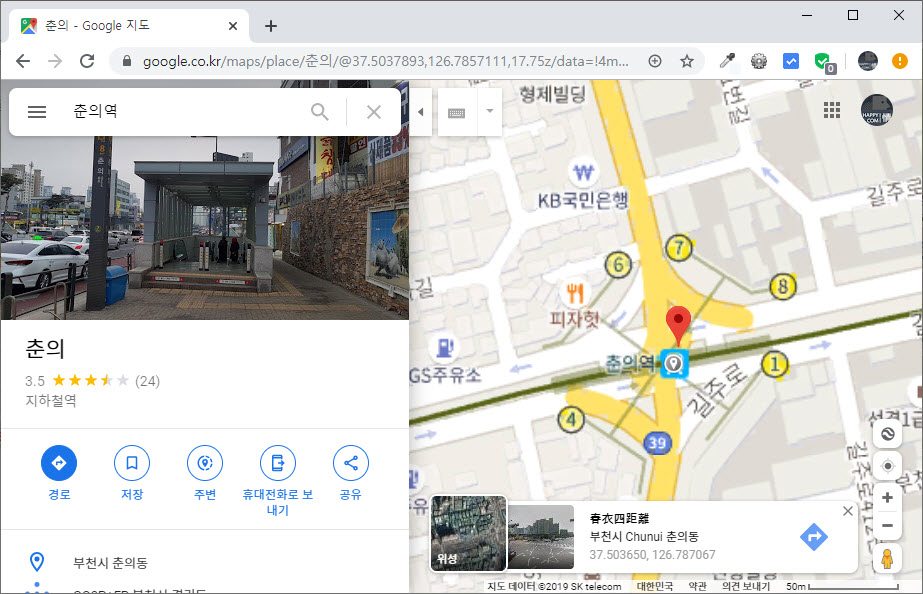
4. 아래의 내용을 복사해서 위의 값으로 좌표를 변경합니다.
아래 예제는 춘의역을 검색한 결과입니다.
<!--
<script src="https://maps.googleapis.com/maps/api/js?key=구글지도API키&sensor=false&language=kr"></script>
// 아래의 것은 해외 공개구글키이므로 변경해서 사용해야 합니다.
-->
<script src="https://maps.googleapis.com/maps/api/js?key=AIzaSyBwlNqAEil52XRPHmSVb4Luk18qQG9GqcM&sensor=false&language=kr"></script>
<script>
function initialize() {
var myLatlng = new google.maps.LatLng(37.503650, 126.787067); // 좌표값
var mapOptions = {
zoom: 16, // 지도 확대레벨 조정
center: myLatlng,
mapTypeId: google.maps.MapTypeId.ROADMAP
}
var map = new google.maps.Map(document.getElementById('map_canvas'), mapOptions);
var marker = new google.maps.Marker({
position: myLatlng,
map: map,
title: "춘의역" // 마커에 마우스를 올렸을때 간략하게 표기될 설명글
});
}
window.onload = initialize;
</script>
<div id="map_canvas" style="width: 100%; height: 400px; margin:0px;"></div>
<!DOCTYPE html>
<html>
<head>
<title>Simple Map</title>
<meta name="viewport" content="initial-scale=1.0">
<meta charset="utf-8">
<style>
#map_canvas {
width : 100%; /* 구글 지도 넓이 */
height: 300px; /* 구글 지도 높이 */
font-size:12px;
}
/* 말풍선관련 css 시작 */
#map_canvas .map_Heading { /* 말풍선 타이틀(회사명) css */
line-height:30px;
font-size:20px;
font-weight:bold;
color:#30C;
}
#map_canvas .map_Content { /* 말풍선 내용 css */
font-size:12px;
color:#333;
}
/* 말풍선 회사홈페이지 링크 css */
#map_canvas a:link.map_Content { text-decoration: none; color: #333; }
#map_canvas a:active.map_Content { text-decoration: none; color: #333; }
#map_canvas a:visited.map_Content { text-decoration: none; color: #333; }
#map_canvas a:hover.map_Content { text-decoration: none; color: #A2002E; }
/* 말풍선관련 css 끝 */
</style>
</head>
<body>
<div id="map_canvas" style="border:1px solid #ccc; margin:0 0 0px 0;"></div>
<script>
/*
API키는 https://developers.google.com/maps/documentation/javascript/get-api-key 에서 발급합니다.
http://maps.googleapis.com/maps/api/geocode/xml?sensor=true&address=서울시 구로구 디지털로 242
위의 링크에서 뒤에 주소를 적어서 웹브라우저로 실행하면 x,y 값을 구할수 있습니다.
<location>
<lat>37.4808224</lat> 이것이 Y_point
<lng>126.8926653</lng> 이것이 X-point
</location>
Sample https://developers.google.com/maps/documentation/javascript/tutorial?hl=ko
*/
var map;
var Y_point = 37.4808224; // lat 값
var X_point = 126.8926653; // lng 값
var zoomLevel = 15; // 첫 로딩시 보일 지도의 확대 레벨
var markerTitle = "해피정닷컴"; // 현재 위치 마커에 마우스를 올렸을때 나타나는 이름
var markerMaxWidth = 300; // 마커를 클릭했을때 나타나는 말풍선의 최대 크기
// 말풍선 내용
var contentString = '<div>' +
'<span class="map_Heading">'+markerTitle+'</span>'+
'<div class="map_Content">'+
'<a href="tel:070-7600-3500" class="map_Content">☎ 070-7600-3500</a> / Fax.02-865-3528<br />메일주소: <a href="mailto:mail@happyjung.com" class="map_Content">mail@happyjung.com</a><br />'+
'서울시 구로구 디지털로 242 한화비즈메트로1차 15층<br />' +
'<a href="https://www.happyjung.com" target="_blank" class="map_Content">https://www.happyjung.com</a>'+
'</div>'+
<!-- // -->
'</div>';
function initMap() {
var myLatlng = new google.maps.LatLng(Y_point, X_point);
var mapOptions = {
zoom: zoomLevel,
center: myLatlng,
mapTypeId: google.maps.MapTypeId.ROADMAP
}
var map = new google.maps.Map(document.getElementById('map_canvas'), mapOptions);
var marker = new google.maps.Marker({
position: myLatlng,
map: map,
draggable:true,
animation: google.maps.Animation.DROP,
title: markerTitle
});
var infowindow = new google.maps.InfoWindow({
content: contentString,
maxWidth: markerMaxWidth
});
infowindow.open(map, marker);
google.maps.event.addListener(marker, 'click', function() {
infowindow.open(map, marker);
});
}
</script>
<script src="https://maps.googleapis.com/maps/api/js?key=API키&callback=initMap" async defer></script>
</body>
</html>
참고자료
http://www.happycgi.com/detail.cgi?number=14849
http://blog.naver.com/PostView.nhn?blogId=fbstar&logNo=100146300148
https://developers.google.com/maps/documentation/javascript/events?hl=ko
http://miwit.kr/b/mw_tip-4705
https://sir.kr/g5_tip/10431
1. https://www.google.co.kr/maps/ 접속
2. 주소 또는 장소명을 검색
표시된 검색결과의 지도에서 원하는 위치를 마우스 클릭 하여 아래와 같이 " 이곳이 궁금한가요? " 클릭
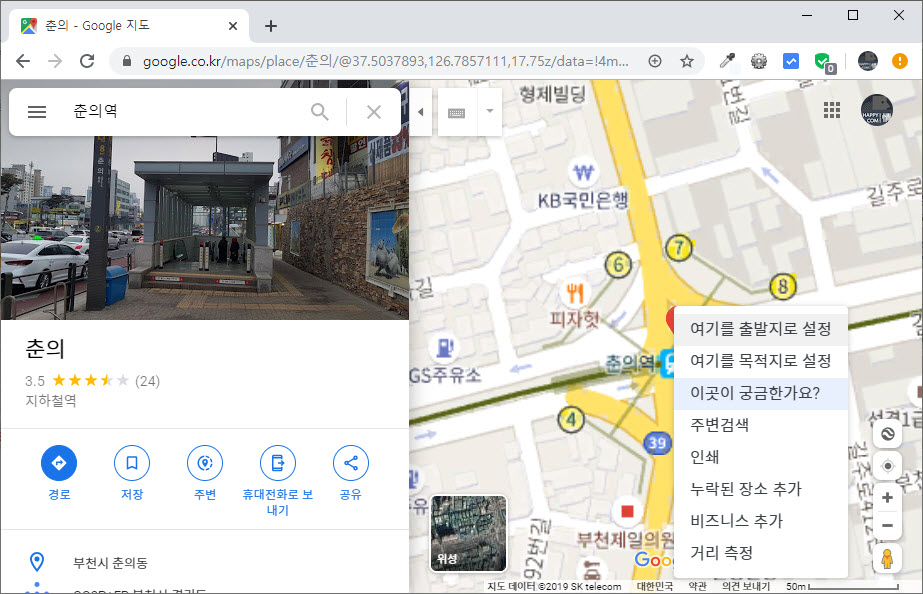
3. 화면 하단의 좌표를 확인합니다.
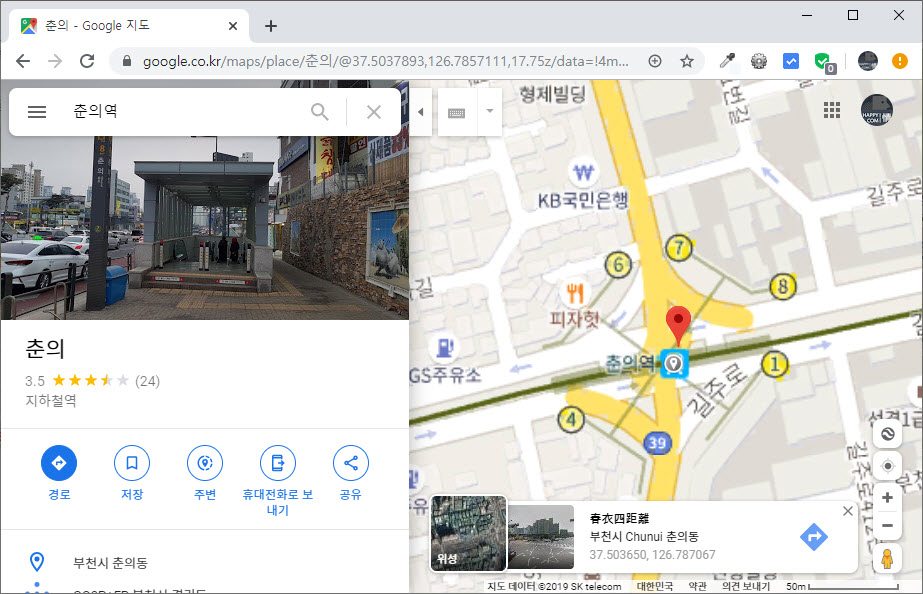
4. 아래의 내용을 복사해서 위의 값으로 좌표를 변경합니다.
아래 예제는 춘의역을 검색한 결과입니다.
<!--
<script src="https://maps.googleapis.com/maps/api/js?key=구글지도API키&sensor=false&language=kr"></script>
// 아래의 것은 해외 공개구글키이므로 변경해서 사용해야 합니다.
-->
<script src="https://maps.googleapis.com/maps/api/js?key=AIzaSyBwlNqAEil52XRPHmSVb4Luk18qQG9GqcM&sensor=false&language=kr"></script>
<script>
function initialize() {
var myLatlng = new google.maps.LatLng(37.503650, 126.787067); // 좌표값
var mapOptions = {
zoom: 16, // 지도 확대레벨 조정
center: myLatlng,
mapTypeId: google.maps.MapTypeId.ROADMAP
}
var map = new google.maps.Map(document.getElementById('map_canvas'), mapOptions);
var marker = new google.maps.Marker({
position: myLatlng,
map: map,
title: "춘의역" // 마커에 마우스를 올렸을때 간략하게 표기될 설명글
});
}
window.onload = initialize;
</script>
<div id="map_canvas" style="width: 100%; height: 400px; margin:0px;"></div>
<html>
<head>
<title>Simple Map</title>
<meta name="viewport" content="initial-scale=1.0">
<meta charset="utf-8">
<style>
#map_canvas {
width : 100%; /* 구글 지도 넓이 */
height: 300px; /* 구글 지도 높이 */
font-size:12px;
}
/* 말풍선관련 css 시작 */
#map_canvas .map_Heading { /* 말풍선 타이틀(회사명) css */
line-height:30px;
font-size:20px;
font-weight:bold;
color:#30C;
}
#map_canvas .map_Content { /* 말풍선 내용 css */
font-size:12px;
color:#333;
}
/* 말풍선 회사홈페이지 링크 css */
#map_canvas a:link.map_Content { text-decoration: none; color: #333; }
#map_canvas a:active.map_Content { text-decoration: none; color: #333; }
#map_canvas a:visited.map_Content { text-decoration: none; color: #333; }
#map_canvas a:hover.map_Content { text-decoration: none; color: #A2002E; }
/* 말풍선관련 css 끝 */
</style>
</head>
<body>
<div id="map_canvas" style="border:1px solid #ccc; margin:0 0 0px 0;"></div>
<script>
/*
API키는 https://developers.google.com/maps/documentation/javascript/get-api-key 에서 발급합니다.
http://maps.googleapis.com/maps/api/geocode/xml?sensor=true&address=서울시 구로구 디지털로 242
위의 링크에서 뒤에 주소를 적어서 웹브라우저로 실행하면 x,y 값을 구할수 있습니다.
<location>
<lat>37.4808224</lat> 이것이 Y_point
<lng>126.8926653</lng> 이것이 X-point
</location>
Sample https://developers.google.com/maps/documentation/javascript/tutorial?hl=ko
*/
var map;
var Y_point = 37.4808224; // lat 값
var X_point = 126.8926653; // lng 값
var zoomLevel = 15; // 첫 로딩시 보일 지도의 확대 레벨
var markerTitle = "해피정닷컴"; // 현재 위치 마커에 마우스를 올렸을때 나타나는 이름
var markerMaxWidth = 300; // 마커를 클릭했을때 나타나는 말풍선의 최대 크기
// 말풍선 내용
var contentString = '<div>' +
'<span class="map_Heading">'+markerTitle+'</span>'+
'<div class="map_Content">'+
'<a href="tel:070-7600-3500" class="map_Content">☎ 070-7600-3500</a> / Fax.02-865-3528<br />메일주소: <a href="mailto:mail@happyjung.com" class="map_Content">mail@happyjung.com</a><br />'+
'서울시 구로구 디지털로 242 한화비즈메트로1차 15층<br />' +
'<a href="https://www.happyjung.com" target="_blank" class="map_Content">https://www.happyjung.com</a>'+
'</div>'+
<!-- // -->
'</div>';
function initMap() {
var myLatlng = new google.maps.LatLng(Y_point, X_point);
var mapOptions = {
zoom: zoomLevel,
center: myLatlng,
mapTypeId: google.maps.MapTypeId.ROADMAP
}
var map = new google.maps.Map(document.getElementById('map_canvas'), mapOptions);
var marker = new google.maps.Marker({
position: myLatlng,
map: map,
draggable:true,
animation: google.maps.Animation.DROP,
title: markerTitle
});
var infowindow = new google.maps.InfoWindow({
content: contentString,
maxWidth: markerMaxWidth
});
infowindow.open(map, marker);
google.maps.event.addListener(marker, 'click', function() {
infowindow.open(map, marker);
});
}
</script>
<script src="https://maps.googleapis.com/maps/api/js?key=API키&callback=initMap" async defer></script>
</body>
</html>
참고자료
http://www.happycgi.com/detail.cgi?number=14849
http://blog.naver.com/PostView.nhn?blogId=fbstar&logNo=100146300148
https://developers.google.com/maps/documentation/javascript/events?hl=ko
http://miwit.kr/b/mw_tip-4705
https://sir.kr/g5_tip/10431
댓글목록
등록된 댓글이 없습니다.